How To Secure APIs From Injection Attacks?
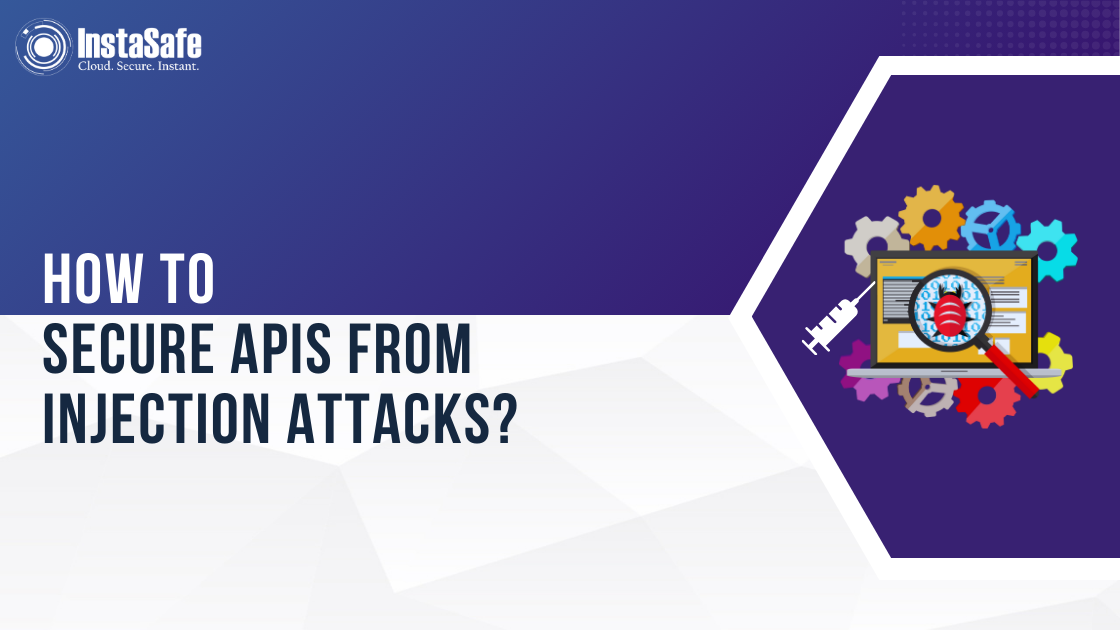
APIs have become crucial for modern software, but their prominence also makes them attractive targets for cybercriminals. Injection attacks, in particular, pose a significant threat to API security.
These attacks exploit vulnerabilities in data processing, potentially leading to unauthorised access, data breaches, or system compromise. This blog will explore how to secure APIs from injection attacks, focusing on key strategies and best practices.
What is an Injection Attack?
Injection attacks occur when malicious code or commands are inserted into API requests. If an API lacks proper security measures, it may execute this malicious input, leading to unauthorised access, data breaches or system compromise.
Injection vulnerabilities arise from inadequate input validation and sanitisation, allowing attackers to exploit how applications process user input.
Types of Injection Attacks
Let’s understand these injection attacks in depth with solutions.
Common Types of Injection Attacks and Ways to Protect Against Them
SQL Injection
SQL injection remains one of the most serious threats to API security. In an SQL injection attack, malicious SQL code is inserted into input fields or parameters used to construct database queries. A successful SQL injection can result in unauthorised data access, modification, or deletion of database contents.
Solution: Use parameterised queries to separate SQL logic from user input. Implement strict input validation and apply the principle of least privilege for database accounts.
Command Injection
Cybercriminals execute arbitrary commands on the host operating system by using a vulnerable program in a command injection attack. This type of injection attack is particularly dangerous as it can lead to complete system compromise.
Solution: Avoid using system commands within applications, opting for language-specific APIs instead. If system commands are necessary, implement strict input validation and whitelisting to ensure only approved commands can be executed.
Cross-Site Scripting (XSS)
While not strictly an injection attack in the traditional sense, XSS is often grouped with injection vulnerabilities. XSS attacks involve injecting malicious scripts into trusted websites, which can then access sensitive information like cookies or session tokens when executed in a victim's browser.
Solution: Always sanitise and encode all user-supplied input before rendering it in web pages. Implement a strong Content Security Policy (CSP) and utilise modern web frameworks with built-in XSS protections.
Securing APIs from Injection Attacks
To protect your APIs from injection attacks, consider implementing the following injection security measures:
Implement Robust Input Validation and Sanitisation
Input validation and sanitisation form the first line of defence against injection attacks. Ensure that all user inputs are thoroughly validated and sanitised before processing:
- Validate Input: Check that incoming data matches the expected format, type and length.
- Sanitise Input: Remove or escape special characters that could be interpreted as code.
- Use Whitelisting: Create a list of allowed inputs rather than trying to blacklist all possible malicious inputs.
Utilise Parameterised Queries
When working with databases, you should never put user input straight into SQL queries. Instead, you should use parameterised queries or prepared statements. This approach separates the SQL logic from the data, making it significantly harder for attackers to inject malicious code.
Example (using Python with psycopg2):
cursor.execute("SELECT * FROM users WHERE username = %s AND password = %s", (username, password))
Implement Strong Authentication and Authorisation
Strong authentication and authorisation mechanisms are important for API injection security:
- Use secure token-based authentication methods like OAuth 2.0 or JWT.
- Implement multi-factor authentication for sensitive operations.
- Apply the concept of least privilege, allowing users just the necessary privileges.
Leverage API Gateways
An API gateway can provide an additional layer of security by:
- Filtering and validating incoming requests
- Implementing rate limiting to prevent brute-force attacks
- Centralising authentication and authorisation processes
Conduct Regular Security Audits and Penetration Testing
Regularly assess your API's security posture:
- Perform security audits to identify potential vulnerabilities.
- Perform regular penetration testing to recreate practical attack situations.
- Use automated scanning tools to detect common injection vulnerabilities.
Implement Proper Error Handling
Avoid exposing detailed error messages to clients, as these can provide valuable information to attackers. Instead, implement proper error handling:
- Use generic error messages for clients.
- Log detailed error information securely for internal debugging and monitoring.
Specific Strategies for SQL Injection Prevention
- Use ORM Libraries: Object-Relational Mapping (ORM) libraries often include built-in protections against SQL injection. Even though they aren't perfect, they can add an extra layer of security.
- Implement Database Least Privilege: Ensure that the database user employed by your application has only the minimum necessary permissions. This can limit the potential damage if an attacker manages to execute malicious SQL.
Specific Strategies for Command Injection Prevention
- Avoid System Calls When Possible: If your application doesn't need to execute system commands, don't. Often, there are safer alternatives that can be implemented entirely within your application's programming language.
- Use Built-in Functions Instead of Shell Commands: Many programming languages offer built-in functions for operations that might otherwise require shell commands. These are generally safer to use.
- Use Shell Escaping Functions: If you must include user input in a shell command, use your programming language's shell escaping function to neutralise special characters.
Lessons from Real-World API Attacks
Instagram API Breach (2019)
This incident exposed personal information of high-profile users due to weak authentication and authorisation mechanisms. It shows how important strong injection security methods and strict access controls are to stop people from getting to data without permission.
Snapchat API Breach (2013)
Exploiting insecure direct object references, this breach allowed attackers to access user data by manipulating API request parameters. It underscores the importance of thorough input validation and parameterised queries in preventing injection attacks.
Uber Data Breach (2016)
This breach, exposing millions of users' data, resulted from weak authentication in a third-party cloud service. It emphasises the need for strong security measures across all API integrations, including those with external services.
Conclusion
Securing APIs from injection attacks is a critical aspect of modern application security. You can make your APIs much less likely to have injection flaws by using a multi-layered method that includes validating input, parameterised searches, strong authentication, and regular security checks.
ZTNA, or Zero Trust Network Access protects your APIs from injection attacks with advanced security features. Our solution validates inputs, uses secure queries, and enforces strong authentication. With InstaSafe, you get a simple, powerful defence against cyber threats, keeping your systems and data safe.
Frequently Asked Questions (FAQs)
- What is the difference between a NoSQL vs. SQL Injection?
SQL injection targets relational databases using malicious SQL queries. NoSQL injection exploits non-relational databases by manipulating query parameters. Both aim to access or manipulate unauthorised data, but use different techniques based on database type.
- Which strategy is best for preventing injection attacks?
Parameterised queries are the most effective strategy. They separate SQL code from user input, preventing malicious code execution. Input validation, escaping special characters, and using ORM frameworks also help protect against injection attacks.
- How to secure an API against injection attacks without authentication?
Implement input validation, use parameterised queries, sanitise user inputs, and employ prepared statements. Limited database rights, stored processes, and frequent system updates and patches reduce vulnerabilities.
- Which method secures REST API endpoints against SQL injection attacks?
Use parameterised queries or prepared statements in your API's data access layer. Also, implement proper input validation and sanitisation, utilise an ORM framework and follow the principle of least privilege for database access.
Key Products
Multi Factor Authentication | Identity And Access Management | ZTNA | Zero Trust Application Access | Secure Enterprise Browser
Key Features
Single Sign On | Endpoint Security | Device Binding | Domain Joining | Always On VPN | Contextual Access | Clientless Remote Access | Device Posture Check
Key Solutions
VPN Alternatives | DevOps Security | Cloud Application Security | Secure Remote Access | VoIP Security